Function that moves the given axes (1-:-32) toward the target position value set with a velocity profile toward trapezoidal time.
Note: The difference with the mva_to_n() function is that it can be called at axis(s) already in motion and the final velocity on the target, also different from zero, can be specified.
|
i32 mva_to_n_v (i32 start, stru_mvgenitp stru [, i32 nDef=1] [, i32 holdFlag=0] [, real fr=1])
|
|
Flag to enable the re-acknowledgement of data (even with moving axes).
The flag is reset when the data is taken
|
|
Structure of type stru_mvgenitp containing motion-related parameters
|
|
Size of the information array (number of axes involved). (optional, default 1)
|
|
Flag to command HOLD status to the mission. (optional, default 0)
|
|
Speed reduction value (0-1). (optional, default 1)
See fr
|
|
Variable containing information about the status of the function (see returns codes).
|
|
-5 [M_ILLEGAL_ARGS]
|
Illegal parameter(acc=0 or illegal axis number)
|
-6 [M_IN_ALARM]
|
Alarm (axis ip already set)
|
6 [M_REACHED]
|
Target reached.
This means that either the direction is 0 and the axis is stationary, or the direction is +1 or -1 and the axis has reached one of the end of strokes
|
14 [M_HOLDED]
|
Motion stopped due to HOLD flag.
It is also returned in cases where the desired axis speed is imposed to 0 or the feedrate is set to zero
|
15 [M_ACCEL]
|
Acceleration in progress
|
16 [M_AT_SPEED]
|
Motion at speed
|
17 [M_DECEL]
|
Deceleration in progress
|
|
|
Rule
|
|
The mission may involve multiple axes (max 32).
They will be linearly interpolated in order to reach their targets all together.
If the target is less than (pos_thr/10) away, RTE considers the space null. So in case the user wishes to make micro advances (< pos_thr/10) he will have to set the variable pos_thr appropriately
|
Example of use:
int fase, restartFlag, esito
stru_mvgenitp m1[2]
motion
if(first_time())
fase = 1
endif
select(fase)
case 1
rr(1000) = 30
rr(1001) = 10
rr(1002) = 10
rr(1003) = 10
rr(1004) = 0
rr(2000) = 100
rr(2001) = 10
rr(2002) = 0
rr(2003) = 10
rr(2004) = 0
restartFlag = true
fase = 2
break
case 2
if(esito = M_REACHED)
fase = 3
endif
break
case 3
rr(1000) = 70
rr(1001) = 10
rr(1002) = 10
rr(1003) = 10
rr(1004) = 0
rr(2000) = 100
rr(2001) = 0
rr(2002) = 0
rr(2003) = 10
rr(2004) = 0
restartFlag = true
fase = 4
break
case 4
if(esito = M_REACHED)
fase = 5
endif
break
case 5
rr(1000) = 100
rr(1001) = 10
rr(1002) = 0
rr(1003) = 10
rr(1004) = 0
rr(2000) = 0
rr(2001) = 10
rr(2002) = 0
rr(2003) = 10
rr(2004) = 0
restartFlag = true
fase = 6
break
case 6
if(esito = M_REACHED)
fase = 7
endif
break
case 7
rr(1000) = 0
rr(1001) = 10
rr(1002) = 0
rr(1003) = 10
rr(1004) = 0
rr(2000) = 0
rr(2001) = 0
rr(2002) = 0
rr(2003) = 10
rr(2004) = 0
restartFlag = true
fase = 8
break
case 8
if(esito = M_REACHED)
fase = 1
endif
break
end_select
m1[0].nAx = 1
m1[0].target = rr(1000)
m1[0].v = rr(1001)
m1[0].vf = rr(1002)
m1[0].acci = rr(1003)
m1[0].accf = rr(1004)
m1[0].accH = max_acc(1)
m1[1].nAx = 2
m1[1].target = rr(2000)
m1[1].v = rr(2001)
m1[1].vf = rr(2002)
m1[1].acci = rr(2003)
m1[1].accf = rr(2004)
m1[1].accH = max_acc(2)
esito = mva_to_n_v(restartFlag, m1[0] , 2, hold, fr)
end_motion
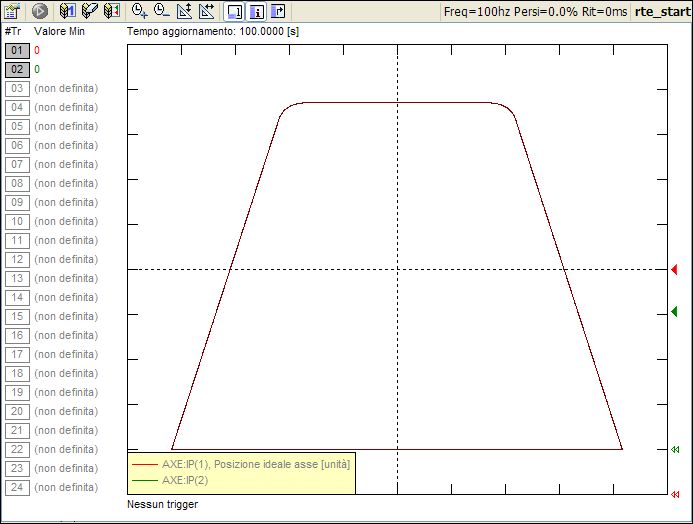
|
Esempio di utilizzo 2:
motion
if(first_time())
fase = 1
endif
if(rise(inp(1)))
fase = 2
m1[0].nAx = 1
m1[0].target = 0
m1[0].v = max_spe(1)
m1[0].vf = 0
m1[0].acci = max_acc(1)
m1[0].accf = 0
m1[0].accH = max_acc(1)
restartFlag = true
endif
if(fase = 1)
iv(1) = ramp(iv(1), 50 + sin(torad(mod(tfb*100, 360))), 10)
else
esito = mva_to_n_v(restartFlag, m1[0], 1, hold, fr)
if(esito = M_REACHED)
fase = 1
endif
endif
end_motion
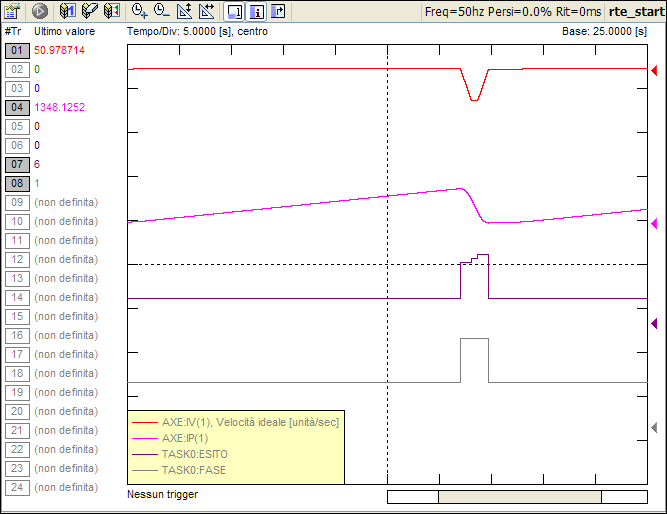
|
|